记录用C++解题的一些基本注意事项(整理柳神笔记+自己遇到的坑)
DevC++的使用
- 让dev支持C++11特性:在编译选项里加上:-std=c++11
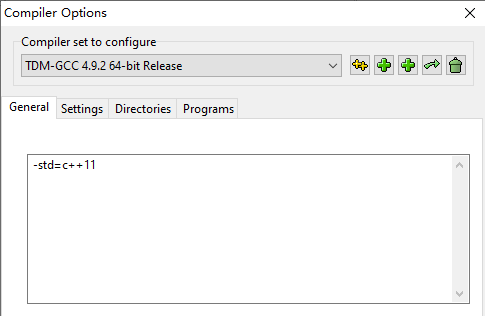
万能头文件:
1 2
| #include<bits/stdc++.h> using namespace std;
|
常用头文件(应对环境限制两手准备):
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| #include <iostream> #include <cstdio> #include <algorithm> #include <cmath> #include <cctype> #include <cstring> #include <vector> #include <set> #include <map> #include <string> #include <stack> #include <queue> #include <bitset> using namespace std;
|
语法注意事项
- gets()禁用,替代品fgets(一个指针,长度,stdin),但是此方法会存入回车,注意长度也多了一个,前面有scanf也要getchar一下;要特别注意scanf(“%s”,a)会将空格作为结束标志
- 10的9次方以内用int,以上用long long
- double scanf中:%lf 输出:%f;long long 两者都是:%lld
- scanf(“%c”,&a)会读取上一次scanf的换行符,所以输入数字后若要再输入字符,应该加一个getchar(),循环中同;即连续输入时,%c会将空格、换行符读入,需要先用getchar接收后面的空格或者换行符,根据需要使用
- n位补码可以表示的数的范围:[-2的n-1次方,2的n-1次方),发生正溢时的范围为:[-2的n-1次方,-2],发生负溢的范围为[0, 2的n-1次方)
- 对于没有指明结束输入的题:用类似while(scanf(“%d”,&a)!=EOF)
- 整数除以二进行四舍五入的操作可以通过判断奇偶数来避免浮点数的介入
- 大的数组,常量定义在主函数之外
- 求绝对值:整数型用abs();浮点型用fabs()
- 如果要读入一整行,也可以用getline():char str[100];cin.getline(str,100);而如果用string容器,则string str;getline(cin,str);
- bool型默认初始化为false,因此bool hash[100]={true};入坑
- stl中是遍历queue和stack,不要用循环,用是否为空
- 特殊的vector需要初始化大小
- 注意memset和fill的区别,一律用fill(arr, arr + n, 要填入的内容);
STL等常规操作
string
1 2 3 4 5
| string s; getline(cin, s); cout << s.length(); string s2 = s.substr(4); string s3 = s.substr(5, 3);
|
vector
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| vector<int> a; cout << a.size() << endl; a.push_back(i); a.pop_back(); vector<int> b(15); vector<int> c(20, 2); for (int i = 0; i < c.size(); i++) { cout << c[i] << " "; } for (auto it = c.begin(); it != c.end(); it++) { cout << *it << " "; } for (auto it : c){ cout << *it << " "; }
vector<int> tin(in, in+n+1);
|
set
1 2 3 4 5 6 7 8 9 10
| set<int> s; s.insert(1); for (auto it = s.begin(); it != s.end(); it++) {
cout << *it << " "; } cout << (s.find(10) != s.end()) << endl;
s.erase(1);
|
map
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| map<string, int> m; m["hello"] = 2; cout << m["hello"] << endl; m["world"] = 3;
for (auto it = m.begin(); it != m.end(); it++) { cout << it->first << " " << it->second << endl; }
cout << m.begin()->first << " " << m.begin()->second << endl;
cout << m.rbegin()->first << " " << m.rbegin()->second << endl;
cout << m.size() << endl;
|
补充:unordered_map (或者unordered_set )省去了排序的过程,如果偶尔刷题时候⽤map 或者set 超时了,可以考虑⽤unordered_map (或者unordered_set )缩短代码运行时间、提⾼代码效率
stack
1 2 3 4 5 6 7
| stack<int> s; for (int i = 0; i < 6; i++) { s.push(i); } cout << s.top() << endl; cout << s.size() << endl; s.pop();
|
queue
1 2 3 4 5 6 7
| queue<int> q; for (int i = 0; i < 6; i++) { q.push(i); } cout << q.front() << " " << q.back() << endl; cout << q.size() << endl; q.pop();
|
位运算bitset
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| bitset<5> b("11");
cout << b << endl; for(int i = 0; i < 5; i++) cout << b[i]; cout << endl << b.any(); cout << endl << b.none(); cout << endl << b.count(); cout << endl << b.size(); cout << endl << b.test(2); b.set(4); b.reset(); b.reset(3); b.flip(); unsigned long a = b.to_ulong();
|
排序问题常用sort()
1 2 3 4 5 6 7 8
| sort(v.begin(), v.end()); sort(arr, arr + 10, cmp); bool cmp(stu a, stu b) { if (a.score != b.score) return a.score > b.score; else return a.number < b.number; }
|
其他常用函数
- isalpha 字⺟(包括⼤写、小写)
- islower (⼩写字母)
- isupper (大写字母)
- isalnum (字母⼤写小写+数字)
- isblank (space和\t )
- isspace ( space 、\t 、\r 、\n )
- tolower (将字母变为小写)
- toupper (将字母变为大写)
- reverse (反转)
- sort(a,a+1000,greater())
C++11新特性
- auto 可以代替⼀大⻓串的迭代器类型声明
- for循环
1 2 3 4 5 6 7 8 9 10 11 12
| int arr[4] = {0, 1, 2, 3}; for (int i : arr) cout << i << endl; int arr[4] = {0, 1, 2, 3}; for (int &i : arr) i = i * 2;
for (auto i : v) cout << i << " ";
for (int i = 0; i < v.size(); i++) cout << v[i] << " ";
|
- to_string()&&string.c_str()
1 2 3 4 5 6
| string s1 = to_string(123); cout << s1 << endl; string s2 = to_string(4.5); cout << s2 << endl; cout << s1 + s2 << endl; printf("%s\n", (s1 + s2).c_str());
|
- 将字符串转化为其他类型
1 2 3 4 5 6 7
| string str = "123"; int a = stoi(str); cout << a; str = "123.44"; double b = stod(str); cout << b; return 0;
|
不不仅有stoi、stod两种,相应的还有:
- stof (string to float)
- stold (string to long double)
- stol (string to long)
- stoll (string to long long)
- stoul (string to unsigned long)
- stoull (string to unsigned long long)
题解注意事项
- 背包问题:注意背包容量边界要取到,背包容积比较为实际值
- 字符串处理:可以考虑正则表达式
- dfs+cin真的可以超时
- 排序传参用引用传参
- 结构体可以通过{}直接构造
常用算法
- 判断素数:
1 2 3 4 5 6 7 8 9 10
| bool isPrime(int a){ if(a <= 1) return false; else{ int q = (int)sqrt(a*1.0); for(int i = 2; i <= q; i++){ if(a % i == 0) return false; } } return true; }
|
- DFS: